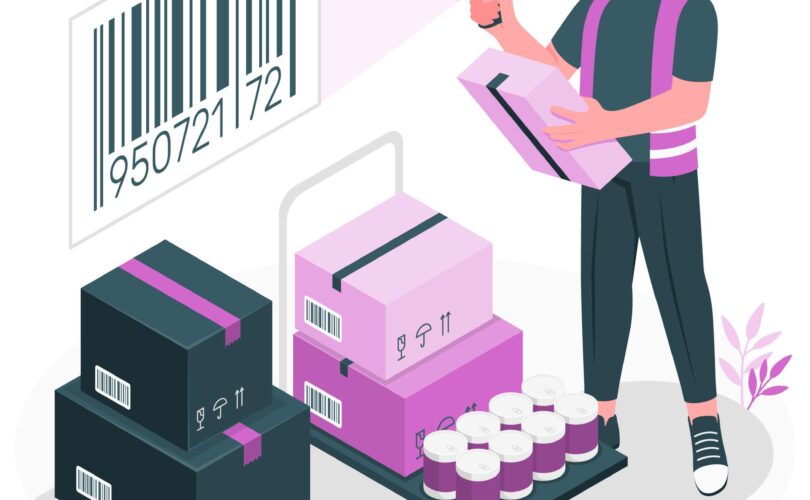
Add barcode scanner to web application
Creating a barcode scanner for a web browser using JavaScript involves a combination of HTML5 features, JavaScript APIs, and possibly external libraries. One common approach is to use the getUserMedia
API to access the user’s webcam and then process the video feed to detect and decode barcodes using a library like QuaggaJS or ZXing. Here’s a basic step-by-step guide to get you started:
Creating a barcode scanner for a web browser using JavaScript involves a combination of HTML5 features, JavaScript APIs, and possibly external libraries. One common approach is to use the getUserMedia API to access the user’s webcam and then process the video feed to detect and decode barcodes using a library like QuaggaJS or ZXing. Here’s a basic step-by-step guide to get you started:
Project Setup:
Create a directory called “barcode-reader” in your server root directory.
HTML Setup:
Just create a HTML file and named it “index.html” in “barcode-reader” and put the given code.
JavaScript Implementation :
Just create a script.js (Javascript) file in “barcode-reader” and put the given code.
Please Note:
This basic implementation will provide you with a functional barcode scanner. However, you might want to enhance the user interface, error handling, and potentially add more features like camera toggling, barcode filtering, or integrating with other parts of your application.
Remember that accessing the webcam requires user permission, and not all browsers support all barcode types.
It can only work on https (SSL) enabled URL.
Additionally, barcode scanning might require good lighting conditions and proper camera focus. Always test thoroughly on various devices and browsers.