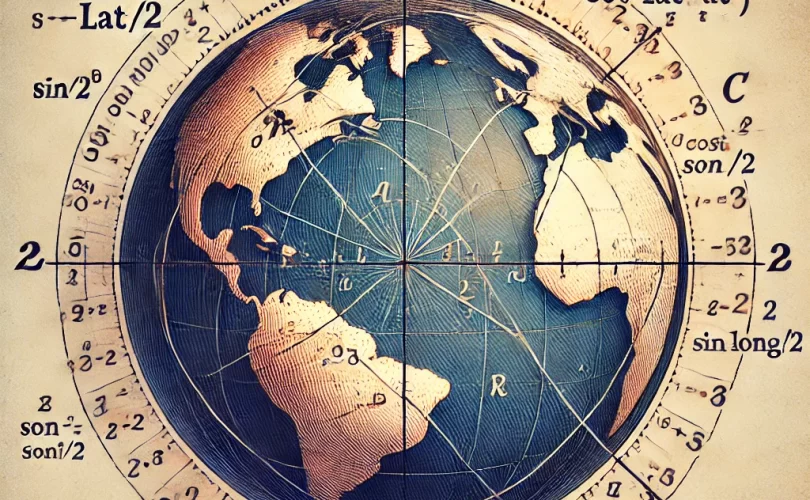
Calculate Distance Between Two Latitude and Longitude Points
Calculating the distance between two geographical locations using their latitude and longitude coordinates is essential for many location-based applications. Whether you’re working with a delivery service, travel app, or simply need to measure distance between cities, PHP provides an easy way to implement this.
In this tutorial, we’ll guide you through the process of calculating the distance between two points using PHP and the Haversine formula, which calculates the shortest distance over the Earth’s surface.
Why Use PHP for Distance Calculation?
PHP is a widely-used server-side scripting language that is great for working with location-based data, especially when building web applications. By calculating the distance between two latitude and longitude points in PHP, you can integrate location functionality into your web app easily.
The Haversine Formula
The Haversine formula calculates the great-circle distance between two points, which is the shortest distance over the Earth’s surface. The formula is:
Where:
- = lat2 – lat1
- = long2 – long1
- = Earth’s radius (mean radius = 6,371 km)
- = Distance between the two points (in kilometers)
PHP Code for Distance Calculation
Here’s a simple PHP function that calculates the distance between two geographical coordinates using the Haversine formula:
How the PHP Code Works:
- Convert Latitude and Longitude to Radians: The function
deg2rad()
converts the latitude and longitude from degrees to radians, as trigonometric functions in PHP require radian values. - Apply the Haversine Formula: The differences between the latitudes and longitudes are calculated, and the Haversine formula is used to find the great-circle distance.
- Return the Distance: The result is returned in kilometers, but you can modify the radius for miles if needed by changing the radius constant.
Conclusion
Calculating the distance between two latitude and longitude points using PHP is straightforward with the Haversine formula. By implementing this method, you can build applications that require accurate distance calculations for geolocation purposes.
Whether you’re developing a GPS-based service, building a travel app, or working with map data, this simple PHP function provides the foundation you need to calculate distances between any two locations on Earth.